JavaScript Theme Switcher
2023.11.05 | Yuki Rea
Do you want to add a light and dark mode to your site or web-app?
There are many ways to implement such a feature, this guide shows you a simple way to implement this using JavaScript and PHP.
Instead of cookies, we will use the localstorage function to store values in the user's browser cache.
We can then read these values to determine what CSS to load later.
The GIF below shows the theme switcher for this site implemented in a similar way to the example in this article.
Go to the Settings page to try it out for yourself.
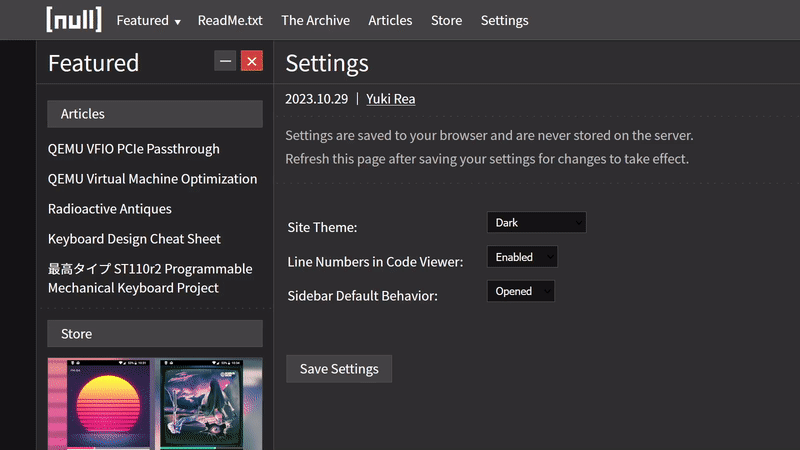
Example Page
You can see the example page I put together below. You can test it out here or open the page in a new tab using the button below.
Render "example.php" in a new tab
Below is the code for example.php which includes all HTML, CSS, PHP, and JavaScript in one page.
The entire page is just 100 lines including comments.
View source of "example.php" in a new tab
example.php
1 <!DOCTYPE html> 2 <html lang="ja" xmlns:og="http://ogp.me/ns#" xmlns:fb="http://ogp.me/ns/fb#"> 3 <meta http-equiv="Content-Type" content="text/html; charset=utf-8"> 4 <head> 5 <meta name="title" content="JavaScript Theme Switcher 6 <meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1" /> 7 <meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=0 "> 8 <title>[null] JavaScript Theme Switcher - EXAMPLE</title> 9 </head> 10 11 12 <!-- CSS styles/themes. --> 13 <style> 14 .dark { 15 background-color: #363636; 16 color: #FFFFFF; 17 } 18 .light { 19 background-color: #f2f2f2; 20 color: #444444; 21 } 22 .high-contrast-dark { 23 background-color: #000000; 24 color: #FFFFFF; 25 } 26 .high-contrast-light { 27 background-color: #FFFFFF; 28 color: #000000; 29 } 30 </style> 31 32 33 <!-- Start body, theme is applied to this element. --> 34 <body> 35 36 37 <!-- Start PHP script to read values from the form. --> 38 <?php 39 // Get the value of "theme" from the form and load it into the $THEME variable. 40 $THEME = htmlspecialchars($_POST['theme']); 41 // If the form was posted and "theme" is set. 42 if (isset($_POST['theme'])) { 43 // Set the value of "theme-example" to localStorage with the content from the "$THEME" variable. 44 echo "<script>localStorage.setItem('theme-example', '$THEME');</script>"; 45 } 46 ?> 47 48 49 <!-- Start JavaScript --> 50 <script> 51 // Get the value of "theme" from localStorage and load it into the "thememode" variable. 52 var thememode = localStorage.getItem("theme-example"); 53 54 // Set the list of themes to the "themes" contant. 55 // This list will be used to clear all applied themes before setting a new theme. 56 const themes = ["dark", "light", "high-contrast-dark", "high-contrast-light"]; 57 58 // Swtich for applying themes. 59 switch(thememode) { 60 case "Dark": 61 document.body.classList.remove(...themes); 62 document.body.classList.add('dark'); 63 break; 64 case "Light": 65 document.body.classList.remove(...themes); 66 document.body.classList.add('light'); 67 break; 68 case "High Contrast Dark": 69 document.body.classList.remove(...themes); 70 document.body.classList.add('high-contrast-dark'); 71 break; 72 case "High Contrast Light": 73 document.body.classList.remove(...themes); 74 document.body.classList.add('high-contrast-light'); 75 break; 76 default: 77 localStorage.setItem('theme-example', 'Dark'); 78 document.body.classList.remove(...themes); 79 document.body.classList.add('dark'); 80 } 81 </script> 82 83 84 <!-- main HTML --> 85 <h1 class=pagename>JavaScript Theme Switcher Example</h1> 86 <!-- Form to select themes, this form posts into the same page it resides in. --> 87 <form action="/posts/javascript-css-settings/example.php" method="POST"> 88 <select id=theme name=theme> 89 <!-- Use PHP and JavaScript to load the currently applied theme into the first drop-down option. --> 90 <option value="<?php echo "<script>document.write(localStorage.getItem('theme-example'));</script>"; ?>"><?php echo "<script>document.write(localStorage.getItem('theme-example'));</script>"; ?></option> 91 <option value"Dark">Dark</option> 92 <option value"Light">Light</option> 93 <option value"High Contrast Dark">High Contrast Dark</option> 94 <option value"High Contrast Light">High Contrast Light</option> 95 </select> 96 <!-- Save and Apply "Submit" button. --> 97 <input id=submit type="submit" value="Save and Apply" /> 98 </form> 99 </body> 100 </html>